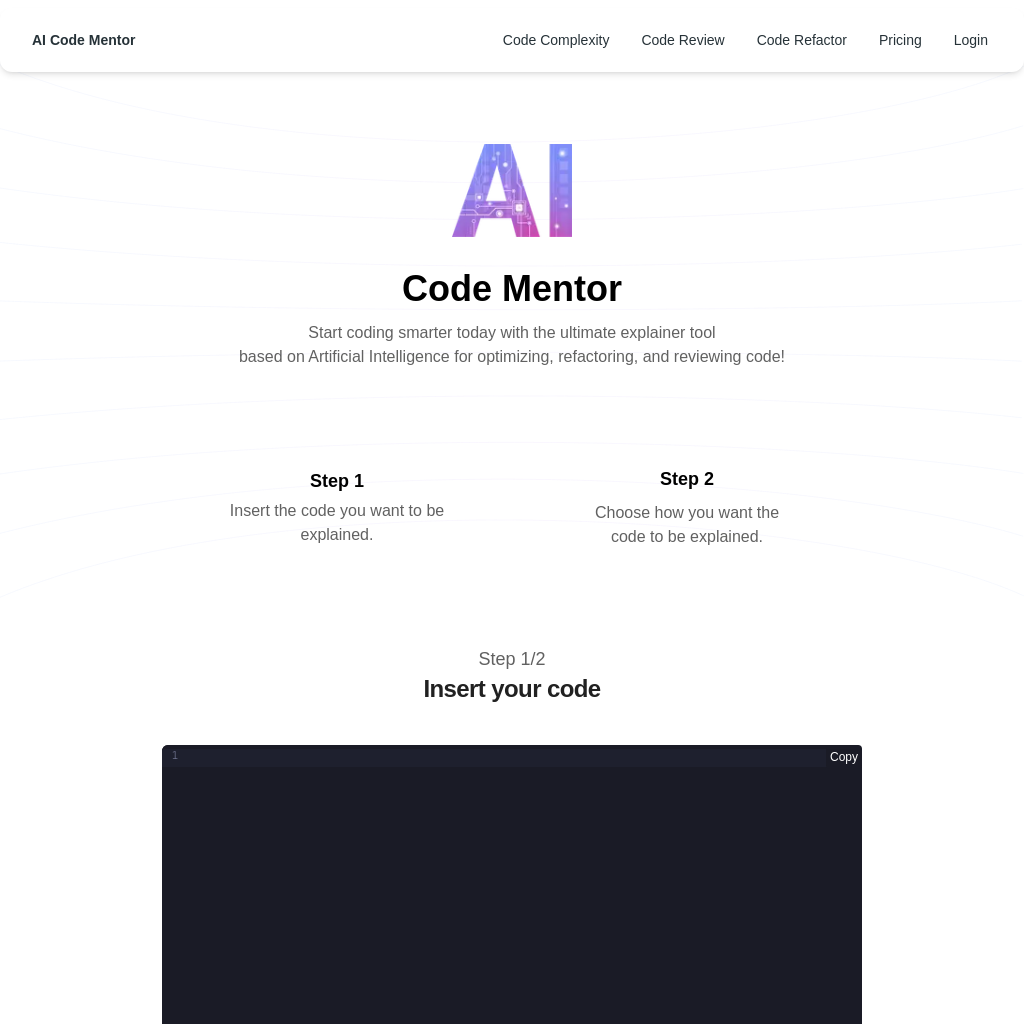
What is AI Code Mentor
This section provides detailed explanations and code implementations of several sorting algorithms. Each algorithm is described with its key steps and logic, helping users understand how they work and how to implement them in code.
How to Use AI Code Mentor
To use these sorting algorithms, simply copy the provided code snippets into your development environment and run them with your desired input array. Each algorithm will sort the array in ascending order.
Features of AI Code Mentor
-
Bubble Sort
A simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
-
Quick Sort
An efficient sorting algorithm that uses a divide-and-conquer approach to sort elements by selecting a pivot and partitioning the array around it.
-
Heap Sort
A comparison-based sorting algorithm that uses a binary heap data structure to sort elements by building a heap and repeatedly extracting the maximum element.
-
Radix Sort
A non-comparative integer sorting algorithm that sorts data by grouping keys by individual digits that share the same significant position and value.